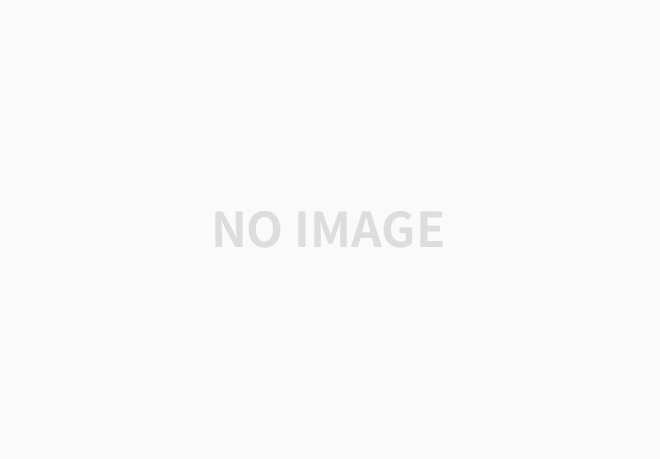
CRC calculation
To compute an n-bit binary CRC, line the bits representing the input in a row, and position the (n+1)-bit pattern representing the CRC's divisor (called a "polynomial") underneath the left-hand end of the row. This is first padded with zeroes corresponding to the bit length n of the CRC. If the input bit above the leftmost divisor bit is 0, do nothing. If the input bit above the leftmost divisor bit is 1, the divisor is XORed into the input (in other words, the input bit above each 1-bit in the divisor is toggled). The divisor is then shifted one bit to the right, and the process is repeated until the divisor reaches the right-hand end of the input row.
void crc_check(uint16_t value, uint8_t crc) {
uint32_t polynom = 0x988000; // x^8 + x^5 + x^4 + 1
uint32_t msb = 0x800000;
uint32_t mask = 0xFF8000;
uint32_t result = (uint32_t)value << 8; // Pad with zeros as specified in spec
while (msb != 0x80) {
// Check if msb of current value is 1 and apply XOR mask
if (result & msb)
result = ((result ^ polynom) & mask) | (result & ~mask);
// Shift by one
msb >>= 1;
mask >>= 1;
polynom >>= 1;
}
}
polynom CRC = x^8 + x^5 + x^4 + 1 의 조합을 생각해서 polynom, msb, mask를 잘 조합하면 될 듯하다.
'Engineer > 소프트웨어 정보' 카테고리의 다른 글
[ISE Design Suite] Multiplexer (0) | 2022.11.20 |
---|---|
ISE Design Suite for Windows 10 - 14.7 설치 & Install (0) | 2022.11.03 |
[STM32] hardware SPI example code (0) | 2020.09.09 |
[STM32F103] delay_us 함수 (0) | 2020.07.24 |
[STM32] Interrupt 사용하기 (0) | 2020.06.24 |